FAQ for HW06 Pennstagram
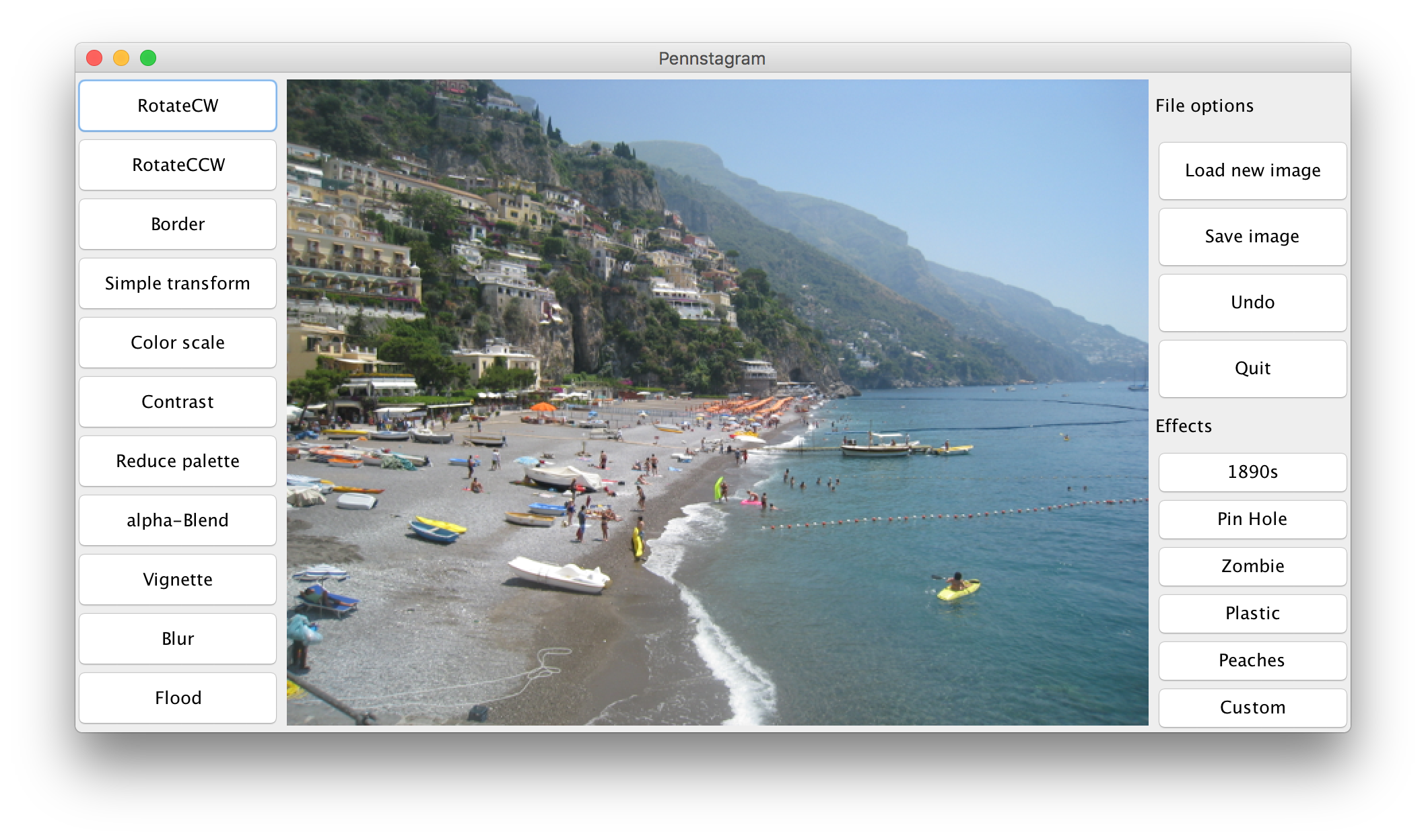
Helpful Links
- HW06 Assignment Page / Instructions
- IntelliJ Setup Guide
- CIS 1200 Java Style Guide
- Course Notes (Java starts @ Chapter 19)
0. How can I make sure I don’t run over the 100 character limit?
Good question! Go to the IntelliJ settings (under “File” on Windows, or “IntelliJ IDEA” on Mac). Go to Editor > Code Style, enter the desired column width in “Visual Guides:”, then click OK. This will create a vertical line so you know approximately where to put line breaks.
Also, the style checker may help you correct lines already over 100 characters.
1. How do I construct a color map?
The ColorMap
class doesn’t have an explicit constructor. If a Java class has
no constructors, an implicit constructor that takes no arguments is
automatically added by the compiler. So you can create a ColorMap
like this:
ColorMap colorMap = new ColorMap();
2. My code has been running for too long. What is wrong?
Performance is mostly beyond the scope of this class, so don’t worry too much
about runtime. Extremely slow code might timeout upon submission, but a few
seconds is within that limit, and our reference solution takes a noticeable
amount of time to finish as well. One common error that causes code to run too
long is making unnecessary copies of the input image to one of your
manipulations. Remember that the getBitmap()
method of PixelPicture
copies
all of the pixels of the PixelPicture
into a new array!
3. The output of one of my methods is just a black rectangle / I am having really unexpected behavior!
Keep in mind throughout this assignment that integer division will truncate the
result. This means that something like 1/1.1
will evaluate to 0
. Make sure
that you are appropriately using doubles where this is an issue. This also may
be indicative of an issue with Pixel
.
4. I made this fancy new workspace, but now I can’t find any of my files.
IntelliJ should show the path to the project root at all times. It looks something like this:
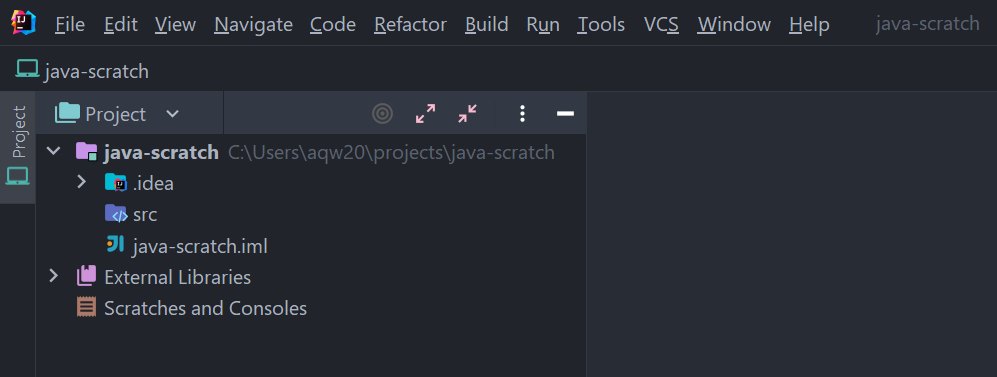
5. My output seems to match the sample images, but I’m failing a lot of tests.
With this assignment, there are a lot of subtle ways to mess up the algorithms that generate an output that looks correct but is ever-so-slightly wrong. One common error is accidentally performing these functions in place. For example, when implementing blur, you should not be modifying the original bitmap as this will lead you to compute subsequent pixels with those you have already blurred. Such small errors could lead to test cases failing, even if the original image looks more or less correct.
6. I am having trouble with my ColorMap
. When I call cmp.put(some pixel, 1)
, the value of some pixel in the map does not increment!
Read the Javadocs for how ColorMap
works. This is not the correct conceptual
understanding of the put function.
7. My image is just a random mess of different colors!
This usually happens because you failed to clip the R
, G
, B
values
generated by one of your manipulations. Make sure you are maintaining that
invariant!
8. How do I implement the getComponents()
method in Pixel
without breaking encapsulation?
Hint: You might find the Arrays.copyOf()
method helpful…
9. Can/should we use helper functions to ensure that the invariant for Pixel is maintained (that values are correctly “clipped”)/in my Blur implementation?
If you find yourself repeating code, and think you can move it to a helper function, go for it!
10. I can’t get my IntelliJ project or tests to run.
Make sure you follow the setup instructions closely.
Are your files in the src or test folder under the org.cis120
package? If you
are using IntelliJ, your project setup should look something like this:
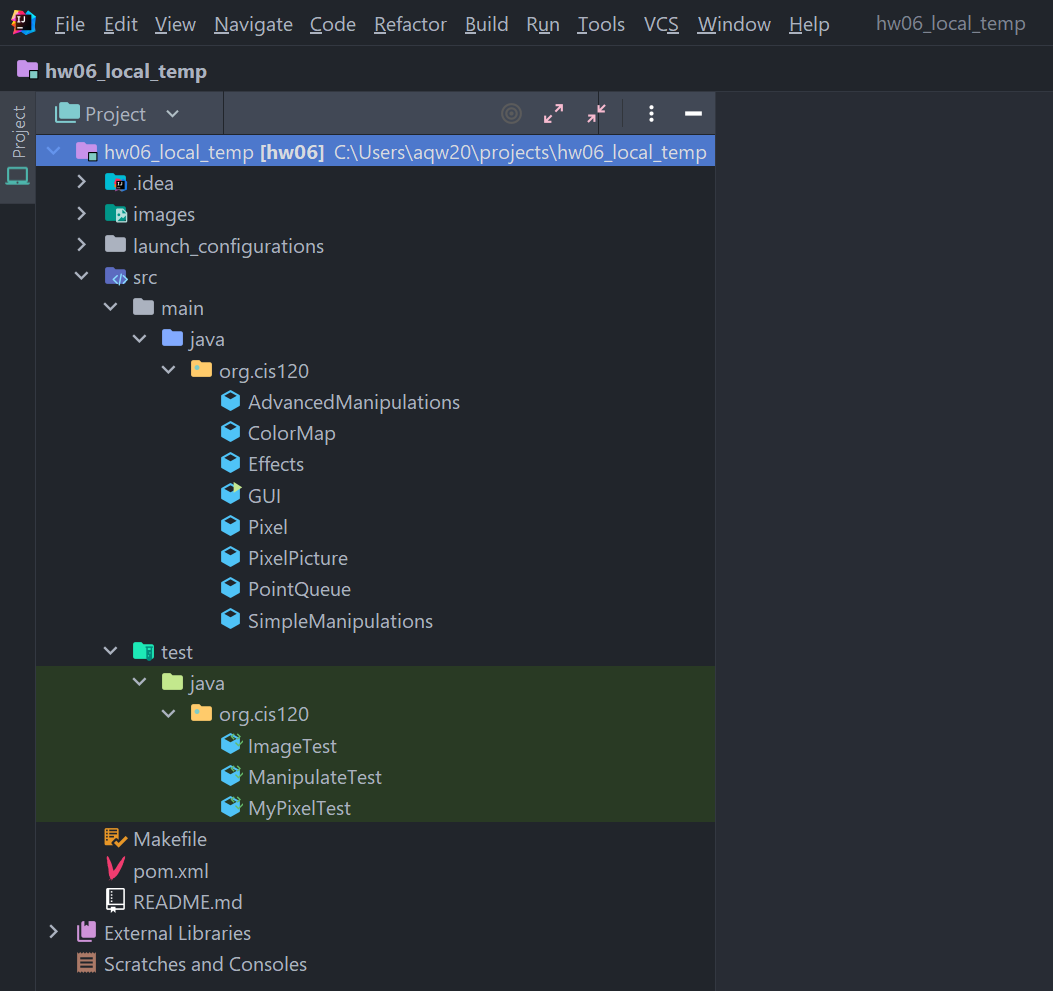